RFont¶
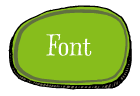
Usage¶
# robofab manual
# Font object
# Usage examples
# start using the current font
from robofab.world import CurrentFont
f = CurrentFont()
# get a clean, empty new font object,
# appropriate for the current environment
f = robofab.world.RFont()
# get an open dialog and start a new font
f = OpenFont()
# open the font at path
f = OpenFont(path)
Description¶
Perhaps the first object you get to play with. The RFont
object is the central part that connects all glyphs with font information (like names, key dimensions etc.). In FontLab the RFont
object talks directly to the glyphs and font data in the FontLab font it belongs to. In UFO or NoneLab use, the RFont
object contains the data and saves it to UFO. RFont
object behave like dictionaries: the glyphname is the key and the returned value is a RGlyph object for that glyph. If the glyph does not exist RFont
will raise an IndexError
.
RFont
has a couple of important sub-objects which are worth checking out. The font’s kerning is stored in a RKerning object and can be reached as an attribute at RFont.kerning
. Fontnames, key dimensions, flags etc are stored in a RInfo object which is available through RFont.info
. The RFont.lib
is an RLib object which behaves as a dictionary.
Iterating¶
One of the most important uses of the RFont
object is that it makes it really easy to iterate (“step through”) the glyphs in the font.
# robofab manual
# Font object
# Iterate through the font object to get to the glyphs.
f = CurrentFont()
for glyph in f:
print glyph.name
This makes the code clear and simple.
FontLab / UFO¶
All basic attributes, methods and behaviour for RFont
objects created in FontLab or in NoneLab are identical. However, the RFont
objects in FontLab have some additional attributes and methods that make special FontLab functionality available. These extra methods and attributes are listed seperately below.
RFont Attributes¶
-
path
¶
The path to the font (read only).
-
kerning
¶
The RKerning object. Cache the font.kerning
object to optimise your script for speed:
# cache the kerning object for speed
from robofab.world import CurrentFont
f = CurrentFont()
cachedKerning = f.kerning
# continue to use cachedKerning, not f.kerning.
-
info
¶
The RInfo object with all the font’s names and key dimensions.
-
lib
¶
The lib object which behaves like a dictionary for arbitrary data that needs to be stored with the font. In FontLab the lib is stored in the .vfb
file. In UFO based fonts the lib is a separate .plist
file. Have a look at how to use the lib.
-
fileName
¶
The filename and path of this font.
-
psHints
¶
A PostScriptFontHintValues object with all font level PostScript hinting information, such as the blue values and stems.
Attribute examples¶
# robofab manual
# Font object
# attribute examples
# Most useful attributes of RFont
# are actually stored in RFont.info
f = CurrentFont()
print f.info.unitsPerEm
# kerning data is available in the kerning object:
print f.kerning
# len() gives you the "length" of the font, i.e. the number of glyphs
print "glyphs in this font:", len(f)
# treat a font object as a dictionary to get to the glyphs
print f["A"]
RFont Methods available in FontLab and UFO¶
-
RFont[glyphName]
Asking the font for a glyph by glyphName
like a dictionary.
-
has_key
(glyphName)¶
Return True
if glyphName
is present in the font.
-
keys
()¶
Return a list of all glyph names in this font.
-
newGlyph
(glyphName, clear=True)¶
Create a new, empty glyph in the font with glyphName
. If clear is True
(by default) this will clear the glyph if it already exists under this name.
Note
clear=True
is now default in both FontLab and NoneLab implementations.
-
removeGlyph
(glyphName)¶
Remove a glyph from the font. This method will show a slightly different behaviour in FontLab and pure Python. In FontLab, components that reference the glyph that is being removed will be decomposed. In plain Python, the components will continue to point to the glyph.
-
insertGlyph
(aGlyph, name=None)¶
Inserts aGlyph
in the font, the new glyph object is returned. If the font already has a glyph with the same name the exisiting data is deleted. The optional as parameter is an alternative glyph name, to be used if you want to insert the glyph with a different name.
Note
As of robofab svn version 200, the as
argument in insertGlyph
has changed to name
. Python2.6+ uses as
as a keyword so it can no longer be used.
-
compileGlyph
(glyphName, baseName, accentNames, adjustWidth=False, preflight=False, printErrors=True)¶
Compile components into a new glyph using components and anchorpoints.
glyphName
- The name of the glyph where it all needs to go.
baseName
- The name of the base glyph.
accentNames
- A list of
accentName
,anchorName
tuples:[('acute', 'top'), etc]
.
-
generateGlyph
(glyphName, replace=True, preflight=False, printErrors=True)¶
Generate a glyph and return it. Assembled from GlyphConstruction.txt
.
replace=True
- The font will replace the glyph if there is already one with this name.
preflight=True
- The font will attempt to generate the glyph without adding it to the font.
Do this to find out if there are any problems to make this glyph. For instance missing glyphs or components could be a problem. See building accents.
-
getReverseComponentMapping
()¶
Get a reversed map of component references in the font:
{
'A' : ['Aacute', 'Aring']
'acute' : ['Aacute']
'ring' : ['Aring']
#etc.
}
-
save
(destDir=None, doProgress=False, saveNow=False)¶
Save the font.
-
autoUnicodes
()¶
Using fontTools.agl
, assign Unicode lists to all glyphs in the font.
-
interpolate
()¶
See how to interpolate for a detailed description of the interpolate method in RFont
.
-
round
()¶
Round all of the coordinates in all of the glyphs to whole integer numbers. For instance a point at (12.3, -10.99)
becomes (12, -11)
. UFO based fonts can deal with floating point coordinates, but for use in FontLab everything needs to be rounded otherwise bad things happen.
-
update
()¶
Call to FontLab to refresh the font. You call update()
after doing lots of manipulating and editing. In UFO based RFont
objects update()
doesn’t do anything, but it exists.
-
copy
()¶
Returns a deep copy of the font, i.e. all glyphs and all associated data is duplicated.
-
getCharacterMapping
()¶
Returns a dict of unicode values to glyph names.
Method examples¶
# robofab manual
# Font object
# method examples
from robofab.world import CurrentFont
f = CurrentFont()
# the keys() method returns a list of glyphnames:
print f.keys()
# find unicodes for each glyph by using the postscript name:
f.autoUnicodes()
FontLab¶
The following attributes and methods are only available to RoboFab objects in FontLab as they’re based on application specific features.
RFont Methods only available in FontLab¶
-
naked
()¶
Return the wrapped fontlab font object itself. This can be useful if you want to set very specific values in the fontlab font that aren’t wrapped or handled by RoboFab objects.
-
writeUFO
(self, path=None, doProgress=False, glyphNameToFileNameFunc=None, doHints=False, doInfo=True, doKerning=True, doGroups=True, doLib=True, doFeatures=True, glyphs=None, formatVersion=2)¶
Write the font to UFO at path.
doProgress=True
- Gives you a progressbar if you want.
glyphNameToFileNameFunc
- An optional callback for alternative naming schemes. See How to use glyph naming schemes.
The other flags are new in RoboFab 1.2 and give you detailed control of what should and should not be written to UFO. The formatVersion
flag determines the format of the UFO, 1
for UFO1, 2
for UFO2.
-
close
()¶
Close the font object and the font window in FontLab.
-
appendHGuide
()¶
Append a horizontal guide.
-
appendVGuide
()¶
Append a vertical guide.
-
clearHGuides
()¶
Clear all horizontal guides.
-
clearVGuides
()¶
Clear all vertical guides.
-
generate
(outputType, path=None)¶
Call FontLab to generate fonts with these parameters and location. Have a look at generate fonts for a more detailed description of this method and how to use it.
RFont Attributes available in FontLab only¶
-
selection
¶
A list of selected glyph names in the font window.
Attribute examples¶
# robofab manual
# Font object
# method examples, available in FontLab
from robofab.world import CurrentFont
f = CurrentFont()
# the keys() method returns a list of glyphnames:
print f.selection
# generate font binaries
f.generate('otfcff')